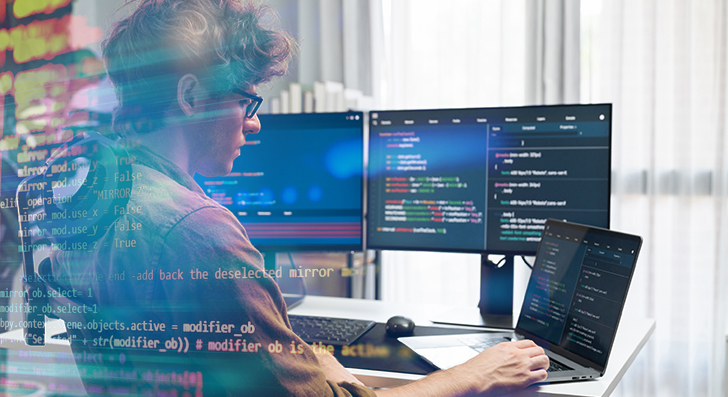
Scalability signifies your software can tackle expansion—far more customers, extra facts, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't something you bolt on later on—it should be portion of your system from the beginning. Quite a few applications fall short when they grow rapidly because the initial design can’t take care of the additional load. As being a developer, you'll want to Believe early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. As an alternative, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale on its own devoid of affecting the whole program.
Also, contemplate your databases from day 1. Will it need to have to manage a million consumers or simply just 100? Pick the suitable type—relational or NoSQL—depending on how your knowledge will improve. Prepare for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t write code that only functions below present-day conditions. Consider what would take place When your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design patterns that help scaling, like concept queues or occasion-driven methods. These assist your app deal with a lot more requests without having finding overloaded.
Any time you Make with scalability in your mind, you are not just planning for fulfillment—you happen to be lessening upcoming problems. A well-prepared procedure is less complicated to keep up, adapt, and expand. It’s greater to organize early than to rebuild later.
Use the Right Databases
Selecting the appropriate database is a vital Component of constructing scalable applications. Not all databases are crafted the exact same, and using the wrong one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your details. Could it be extremely structured, like rows inside of a table? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like read replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally more simply.
Also, consider your go through and produce designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can manage high create throughput, as well as celebration-based information storage programs like Apache Kafka (for momentary details streams).
It’s also smart to Feel forward. You might not need Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases performance when you mature.
In short, the right database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll help save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away anything at all unneeded. Don’t choose the most complex Option if an easy one will work. Maintain your functions small, targeted, and straightforward to test. Use profiling tools to find bottlenecks—destinations in which your code takes far too extended to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually gradual points down greater than the code alone. Make certain Each individual query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across massive tables.
For those who recognize the exact same data getting asked for again and again, use caching. Retail outlet the results briefly working with applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and will make your app much more productive.
Make sure to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to handle 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to handle much more end users plus much more website traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment support keep the application rapidly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of a person server executing every one of the operate, the load balancer routes consumers to various servers based on availability. This suggests no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for the exact same details again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two frequent different types of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to website the person.
Caching minimizes databases load, improves pace, and will make your application more productive.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are simple but effective instruments. Together, they help your app manage additional customers, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess upcoming potential. When traffic increases, you are able to include much more sources with only a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. It is possible to give attention to creating your app rather than managing infrastructure.
Containers are A further vital Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This can make it effortless to move your application between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
When your application works by using a number of containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be simple to different areas of your application into expert services. You are able to update or scale pieces independently, that's great for functionality and reliability.
Briefly, utilizing cloud and container applications implies you could scale rapidly, deploy easily, and Recuperate quickly when troubles happen. If you prefer your app to improve with out boundaries, start employing these applications early. They conserve time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t keep track of your software, you received’t know when things go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make greater selections as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your app way too. Control just how long it will require for people to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Build alerts for vital complications. Such as, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified promptly. This will help you resolve concerns quick, frequently before buyers even detect.
Checking is additionally helpful when you make improvements. When you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back prior to it results in authentic injury.
As your app grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of problems until it’s far too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a powerful Basis. By designing meticulously, optimizing wisely, and using the suitable resources, you may build apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Make wise.